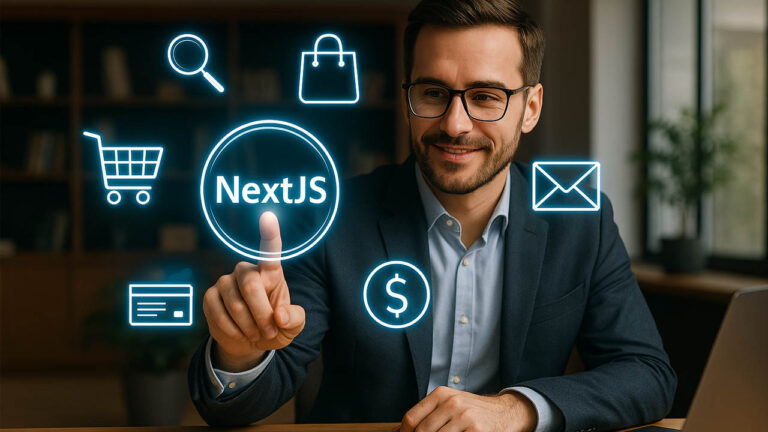
Picture this: you’re shopping online for a new pair of sneakers. The page loads lightning-fast with a sleek image gallery, but as you scroll, the reviews section stutters, the “Add to Cart” button lags, and suddenly you’re wondering if the site’s worth your time. Speed matters in e-commerce—every second of delay can cost sales. Enter NextJS, the React framework that’s become a darling of web developers, and its secret weapon: partial hydration. It’s a technique that promises to keep product pages snappy, interactive, and delightful without drowning users in heavy JavaScript.
Table of Contents
In this article, we’ll dive into partial hydration in NextJS, focusing on how it can supercharge e-commerce product pages. We’ll unpack what it is, why it’s a game-changer, and roll up our sleeves with advanced techniques to make it work in the real world. Whether you’re a seasoned developer or just dipping your toes into NextJS, this guide will give you practical ideas to build faster, smarter online stores. Let’s get started.
What Is Partial Hydration?
Hydration in web development is like waking up a static page and giving it life. Traditionally, a server-rendered React app sends HTML to the browser, then JavaScript kicks in to make it interactive—a process called full hydration. It’s powerful but can be overkill. Load a product page with a carousel, reviews, and a chat widget, and you’re shipping a ton of JavaScript that executes all at once, bogging down performance.
Partial hydration flips that script. With NextJS, you can choose which parts of a page get hydrated—and when. The static stuff (like product descriptions or images) stays as lightweight HTML, while interactive bits (like buttons or forms) get JavaScript only when needed. It’s like serving a meal in courses instead of dumping the whole buffet on the table at once.
NextJS introduced experimental support for partial hydration around version 12, and it’s been evolving fast. By 2025, with frameworks and tools maturing, it’s a must-know for e-commerce devs chasing Google’s Core Web Vitals and happier customers.
Why Partial Hydration Matters for E-commerce
E-commerce product pages are a balancing act. They need to be fast (to hook shoppers), rich (to showcase products), and interactive (to seal the deal). Partial hydration nails all three. Here’s why it’s a big deal:
Speed Wins Sales
Studies like Google’s show that a 0.1-second improvement in load time can boost conversions by 8%. Partial hydration slashes initial JavaScript payloads, letting pages render faster—crucial for that first impression on a product page.
Mobile Matters
Half of online shopping happens on phones, often over shaky connections. Sending less JavaScript upfront means mobile users get a usable page sooner, not a blank screen while the browser chugs.
SEO and UX Harmony
Search engines love fast, server-rendered content. Shoppers love smooth interactions. Partial hydration delivers both: static HTML for crawlers, targeted hydration for users.
Scalability
For a store with thousands of products, fully hydrating every page is a resource hog. Partial hydration scales better, keeping server costs down and performance up.
How Partial Hydration Works in NextJS
Before we get fancy, let’s break down the basics. NextJS leans on Server-Side Rendering (SSR) and Static Site Generation (SSG) to deliver HTML. Partial hydration builds on that by letting you defer or skip hydration for specific components. Here’s the flow:
- Server Renders the Page: NextJS generates HTML for the product page—title, images, description—using SSG or SSR.
- Client Gets Selective JS: Instead of hydrating the whole page, NextJS sends only the JavaScript needed for interactive components (e.g., a quantity picker).
- Lazy Hydration Kicks In: Other parts hydrate on demand—like when a user scrolls to reviews or clicks a tab.
NextJS makes this possible with features like next/dynamic for lazy-loading and experimental flags like react-root (in older versions) or built-in optimizations in newer releases. Now, let’s level up with some advanced techniques tailored for e-commerce.
Advanced Techniques for E-commerce Product Pages
Building a product page with partial hydration isn’t just about slapping dynamic imports everywhere. It’s about strategy—knowing what to hydrate, when, and how. Here are some battle-tested approaches:
1. Prioritize Critical Interactions
Not every component needs to wake up right away. Focus on what drives sales:
Add to Cart Button: Hydrate this immediately. It’s the money-maker. Use a simple client-side component:
import { useState } from 'react'; export default function AddToCart() { const [count, setCount] = useState(1); return ( <button onClick={() => setCount(count + 1)}> Add to Cart ({count}) </button> ); }
Product Images: Keep these static. A <picture> tag with lazy-loaded <img> elements works fine without JS.
Reviews Section: Defer hydration until the user scrolls into view (more on that below).
2. Lazy-Load with next/dynamic
NextJS’s dynamic import is your best friend for delaying hydration. For a reviews widget that only loads when visible:
import dynamic from 'next/dynamic';
const Reviews = dynamic(() => import('../components/Reviews'), {
ssr: false, // Skip server rendering
loading: () => <p>Loading reviews...</p>,
});
export default function ProductPage() {
return (
<div>
<h1>Super Sneakers</h1>
<img src="/sneakers.jpg" alt="Sneakers" loading="lazy" />
<AddToCart />
<Reviews />
</div>
);
}
Here, Reviews doesn’t hydrate until the browser needs it, cutting initial load time.
3. Intersection Observer for Scroll-Based Hydration
For components lower on the page—like related products or Q&A—use the Intersection Observer API to hydrate only when they’re in view. Here’s a custom hook:
import { useEffect, useRef, useState } from 'react';
import dynamic from 'next/dynamic';
const RelatedProducts = dynamic(() => import('../components/RelatedProducts'), {
ssr: false,
});
function useOnScreen() {
const ref = useRef();
const [isVisible, setIsVisible] = useState(false);
useEffect(() => {
const observer = new IntersectionObserver(
([entry]) => setIsVisible(entry.isIntersecting),
{ threshold: 0.1 }
);
if (ref.current) observer.observe(ref.current);
return () => observer.disconnect();
}, []);
return [ref, isVisible];
}
export default function ProductPage() {
const [ref, isVisible] = useOnScreen();
return (
<div>
<h1>Super Sneakers</h1>
<AddToCart />
<div ref={ref}>
{isVisible ? <RelatedProducts /> : <p>Scroll to see more...</p>}
</div>
</div>
);
}
This keeps the payload lean until the user scrolls, perfect for long product pages.
4. Island Architecture
Borrowing from frameworks like Astro, “islands” are isolated interactive zones in a sea of static content. Split your product page into islands:
- Island 1: Add to Cart and quantity selector.
- Island 2: Variant picker (color, size).
- Static Sea: Description, specs, images.
Each island hydrates independently. Use next/dynamic with a wrapper to mark islands:
const VariantPicker = dynamic(() => import('../components/VariantPicker'), {
ssr: false,
});
export default function ProductPage({ product }) {
return (
<main>
<h1>{product.name}</h1>
<p>{product.description}</p>
<VariantPicker variants={product.variants} />
<AddToCart />
</main>
);
}
export async function getStaticProps({ params }) {
const product = await fetchProduct(params.id); // Your API call
return { props: { product } };
}
This keeps the page lightweight while ensuring interactivity where it counts.
5. Preload Critical JS with <Script>
For must-have interactions (like Add to Cart), use NextJS’s <Script> component to preload JavaScript without blocking the render:
import Script from 'next/script';
export default function ProductPage() {
return (
<>
<Script
src="/add-to-cart.js"
strategy="afterInteractive"
/>
<h1>Super Sneakers</h1>
<div id="cart-root" />
</>
);
}
Pair this with a tiny client-side script to hydrate the cart, keeping the main bundle slim.
Real-World Example: A Sneaker Product Page
Let’s tie it together with a sample. Imagine a sneaker page with:
- A static hero image and title (SSG).
- An Add to Cart button (hydrated on load).
- A reviews section (hydrated on scroll).
import dynamic from 'next/dynamic';
import { useEffect, useRef, useState } from 'react';
const Reviews = dynamic(() => import('../components/Reviews'), { ssr: false });
const AddToCart = dynamic(() => import('../components/AddToCart'), { ssr: false });
function useOnScreen() {
const ref = useRef();
const [isVisible, setIsVisible] = useState(false);
useEffect(() => {
const observer = new IntersectionObserver(
([entry]) => setIsVisible(entry.isIntersecting),
{ threshold: 0.1 }
);
if (ref.current) observer.observe(ref.current);
return () => observer.disconnect();
}, []);
return [ref, isVisible];
}
export default function ProductPage({ product }) {
const [ref, isVisible] = useOnScreen();
return (
<main>
<h1>{product.name}</h1>
<img src={product.image} alt={product.name} loading="lazy" />
<p>{product.description}</p>
<AddToCart productId={product.id} />
<div ref={ref}>
{isVisible ? <Reviews productId={product.id} /> : <p>Loading reviews...</p>}
</div>
</main>
);
}
export async function getStaticProps({ params }) {
const product = await fetchProduct(params.id); // Mock API
return { props: { product } };
}
export async function getStaticPaths() {
const products = await fetchAllProducts(); // Mock API
const paths = products.map((p) => ({ params: { id: p.id } }));
return { paths, fallback: 'blocking' };
}
This setup delivers a fast initial render, hydrates the cart instantly, and loads reviews only when needed. Add a CDN like Vercel, and you’ve got a zippy page ready for thousands of shoppers.
Challenges and Trade-Offs
Partial hydration isn’t a silver bullet. Here’s what to watch out for:
Complexity
Splitting hydration adds code overhead. You’ll need to plan components carefully and debug edge cases—like a lazy-loaded section failing to hydrate on slow networks.
SEO Nuance
Search engines are fine with static content, but if key info (like pricing) is in a deferred component, you might need extra SSR tricks to ensure it’s crawlable.
User Perception
A loading spinner for reviews might feel janky if it takes too long. Balance delays with placeholders or skeletons to keep the experience smooth.
The Future of Partial Hydration in E-commerce
NextJS is pushing the envelope—version 14 and beyond might bake partial hydration deeper into the framework, with better tools and less boilerplate. For e-commerce, this could mean product pages that load in milliseconds, adapt to user behavior, and scale effortlessly. Pair it with edge computing (like Vercel’s Edge Functions) or AI-driven personalization, and you’ve got a recipe for next-level shopping.
So, next time you’re building a product page, think partial. Hydrate what matters, defer the rest, and watch your metrics—and your customers—thank you. Have you tried partial hydration yet?